Conditions
In dialog designer, conditions can be used either in an action dialog element or a branch dialog element. A typical example would be, you accept some input from the user, and based on its value you want to branch to a particular dialog. A condition necessarily evaluates either True or False. A condition that evaluates True causes an action dialogue element to do the action, and a condition that evaluates True for a branch dialogue element to invoke the dialogue. Since there will usually be more than one condition in each of the two dialogue components, the order in which you specify the conditions is crucial. Subsequent conditions are not evaluated if a condition is matched or evaluated to be true. Consider the scenario where your chatbot only supports English, French, and German and you want to go back to English if the user doesn't prefer either of those two languages. Based on the value of a variable, such as $conv.lang, you would call a dialogue that greets the user in the proper language. If the user's preferred language is neither German nor French, your last condition will be True, as seen in the image below. You want to launch the relevant dialogue if the user’s language is either German or French.
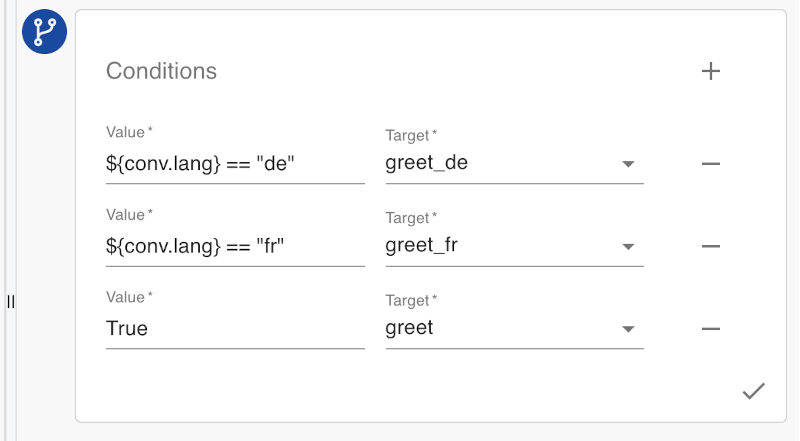
If the last condition shown in the image above is put as the first, no matter what the value of ${conv.lang} is, greet dialog will always be invoked.
Conditions can be written by writing single-line expressions which can be formed by using the below-supported constructs.
Boolean Constants
The following boolean constants are supported:
- True
- False
The boolean constant True has already been used in the example above to demonstrate how it might be helpful to initiate an action or branch to a dialogue regardless of the fallback situation.
Strings, Numbers, and Variables
- Anything included in double quotes is a valid string. Strings in a single quote are not supported.
- Numbers have to start with a digit and if they have a decimal point there has to be at least one digit after that.
- Every variable name has to start with ${ and has to end with }. In addition, as variables are tied to either (1) User or (2) Conversation or (3) Dialog state, every variable name has to start with ${user.} or ${conv.} or {dialog.dialog_id.}. Variable in the dialog state also is present in the context of a specific dialog; dialog_id captures the id of the dialog. Except for some variables which are set behind the scenes like {conv.__utterance__}, rest of the variables are defined by the users in the dialog designer. Variables can evaluate either to a string or a number.
Strings: "AutomationEdge", "123", "1,234**()"
Numbers: 100, 2.3, 999, 222.50
Variables: ${user.name}, ${dialog.welcome.input1}, ${conv.lang}
Strings: 'AIStudio' (string in single quotes), "AutomationEdge (string without a closing quote)
Numbers: 2., .99 (numbers missing a digit after and before the decimal point respectively), 1.2E10 (number in scientific notation)
Variables: ${foo}, ${foo.bar}, ${dialog.var1} (first two are variables neither in a user nor in a conversation nor in a dialog context, last one is a variable in a dialog context but doesn't have a dialog_id)
Comparison Operators
The following comparison operators are supported:
- ==
- !=
- <
- <=
- >
- >=
All these operators have their usual meanings. Some of the valid conditions using comparison operators are ${conv.lang} == "de", ${conv.d1.salary} <= 10000. Both the operands provided to any of these comparison operators should either be strings or numbers. Of course, you can compare a string with a variable as long as that variable holds a string value.
Boolean Operators
The following boolean operators are supported
- and
- or
- not
Operators "and" and "or" require two operands, one before and one after them, whereas operator "not" only requires one operand, that which comes after it. A boolean constant or an expression that evaluates to a boolean value must be the only operand for these operators. Condition expression "False and False or True" shows the use of these operands which evaluates to True. First False and False is executed which results in False and then False or True is executed which results in True. In a way, evaluation is left to right.
Condition expression "not False and False or True" also evaluates to True, its evaluation proceeds as shown below# Original expression
not False and False or True
# 'not' is applied to False which makes it True, so the expression is equivalent to
True and False or True
# True and False evaluate to False, so the resulting expression is
False or True
# False or True evaluate to True
True
Parentheses
Parentheses or round brackets can be used in an expression to control the order of evaluation of the boolean operators. We will again consider examples from the previous section and see how their evaluation gets affected by the use of parentheses.
- "False and False or True" evaluates to True, but
"False and (False or True)" evaluates to False as with parentheses 'or' condition is evaluated first. - "not False and False or True" evaluates to True, but
"not(False and False or True)" evaluates to False as operand of 'not' in this case is the entire expression which in turn evaluates to True.
Special Functions
The following special functions are supported • contains Accepts two string arguments, and returns True if the first argument completely contains the second argument. contains("ab", "a") would return True but contains("ab" "ba") or contains("ab", "abc") would return False. • startswith Accepts two string arguments, and returns True if the first string has a second string at its start. startswith("abc", "ab") would return True but startswith("abc", "abcd") would return False. • endswith Accepts two string arguments, and returns True if the first string has a second string at its end. endswith("abc", "bc") would return True but endswith("abc", "abcd") would return False. • lower Accepts only one string argument, and returns a string that has all the characters converted to lowercase. • upper Accepts only one string argument, and returns a string which has all the characters converted to uppercase. • len Accepts only one string argument, returns length of the string as a number. • bool converts the state variable to Boolean. Eg., bool(${dialog.welcome.id})